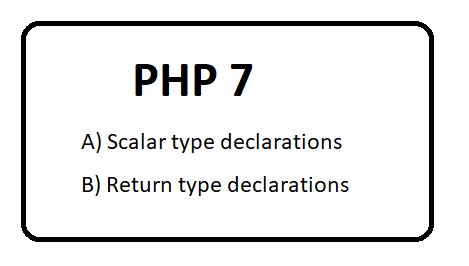
What is a Scalar type declarations?
Allowing/Not-Allowing datatype declaration in functions is known as Scalar type declarations.
Question: What are different types of Scalar type declarations?
1) Coercive Mode (default)
2) Strict Mode
What are different type of data-type can be used in Scalar type declarations?
- int
- float
- bool
- string
- interfaces
- array
- callable
Question: Give the examples of Scalar type declarations with Coercive Mode (default)
Example 1
$a=1; // integer $b=2; //integer function FunctionName1(int $a, int $b){ return $a + $b; } echo FunctionName1($a, $b); //3
Example 2
function sum(int ...$ints) { return array_sum($ints); } print(sum(3, '2', 5.1)); //10
Example 3
function FunctionName3(int $a, int $b){ return $a * $b; } echo FunctionName3('hello', 10); //PHP Fatal error: Uncaught TypeError: Argument 1 passed to FunctionName() must be of the type integer, string given.
Example 4
$a='1One'; $b=2; //integer function FunctionName3(int $a, int $b){ return $a + $b; } echo FunctionName3($a, $b);//RESULT: PHP Notice: A non well formed numeric value encountered on line 5
Question: Give the examples of Scalar type declarations with STRICT MODE?
Example 1
declare(strict_types=1); $a=1; // integer $b=2; //integer function phpFunction (int $a, int $b){ return $a + $b; } echo phpFunction1($a, $b);//3
Example 2
declare(strict_types=1); $a='1'; // String $b=2; //integer function phpFunction1 (int $a, int $b){ return $a + $b; } echo phpFunction1($a, $b);//Fatal error: Uncaught TypeError: Argument 1 passed to phpFunction1() must be of the type int, string given
Question: Give the examples of Return type declarations with STRICT MODE?
Example 1
$a='10'; // string $b=2; // integer function phpFunctionReturn ($a, $b) : int { return $a * $b; } echo phpFunctionReturn ($a, $b);
Example 2
declare(strict_types=1); $a='10'; // string $b=2; // integer function phpFunctionReturn ($a, $b) : string { return $a * $b; } echo phpFunctionReturn ($a, $b);//PHP Fatal error: Uncaught TypeError: Return value of phpFunctionReturn() must be of the type string, integer returned in /home/cg/root/7035270/main.php:6